how to create a webserver That accepts an http POST request that would then write to the same CSV.
Thanks to my boss whose been teaching me nodejs for years now im finally familliar with POST request.
In this post we will create a websocket that will accepts an http POST requestthen write to CSV file in a nonede stream.
POST is how we send data to a server, generally putting the content in the body of the request.
First create a directory of your project, change to it and run npm init
. Then install express as a dependency npm install express --save
add your hallo world default code :
|
const express = require('express')
const app = express()
const port = 3000
app.get('/', (req, res) => {
res.send('Hello World!')
})
app.listen(port, () => {
console.log(`Example app listening at http://localhost:${port}`)
})
|
to test if your sever is working run node fileName.js
Next step will create a node write and read stream funtional modules using writeToStream
a fast-csv
module.
1
2
3
4
5
6
7
8
9
10
11
12
|
const { writeToStream } = require('@fast-csv/format');
const fs = require('fs');
const csv = "./file.csv";
async function appendData(rows) {
const stream = fs.createWriteStream(csvFile, { flags: 'a' })
await stream.write('\n');
await writeToStream(stream, rows);
}
//make functional reusable global
exports.appendData = appendData;
|
be sure to check how writeTosream work
Then the final part we are going to accept POST resquest in out webserver by tweaking our server code .
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
|
const express = require('express')
const app = express()
const port = 3000
//import your node stream funtion
const csvPost = require('<your node stream file>')
// add boddy-paser
const bodyParser = require('body-parser')
const JSONParse = bodyParser.json({ type: 'application/json' });
app.post('/user', JSONParse, (req, res) => {
console.log(req.body);
const entries = []
let log = [
req.body.firstname,
req.body.lastname,
]
entries.push(log)
//call your writeTo stream function
csvAppend.append(entries);
res.sendStatus(200);
});
app.listen(port, () => {
console.log(`listening at http://localhost:${port}`)
})
|
run your server node fileName.js
now the last part will test our POST request via postman.
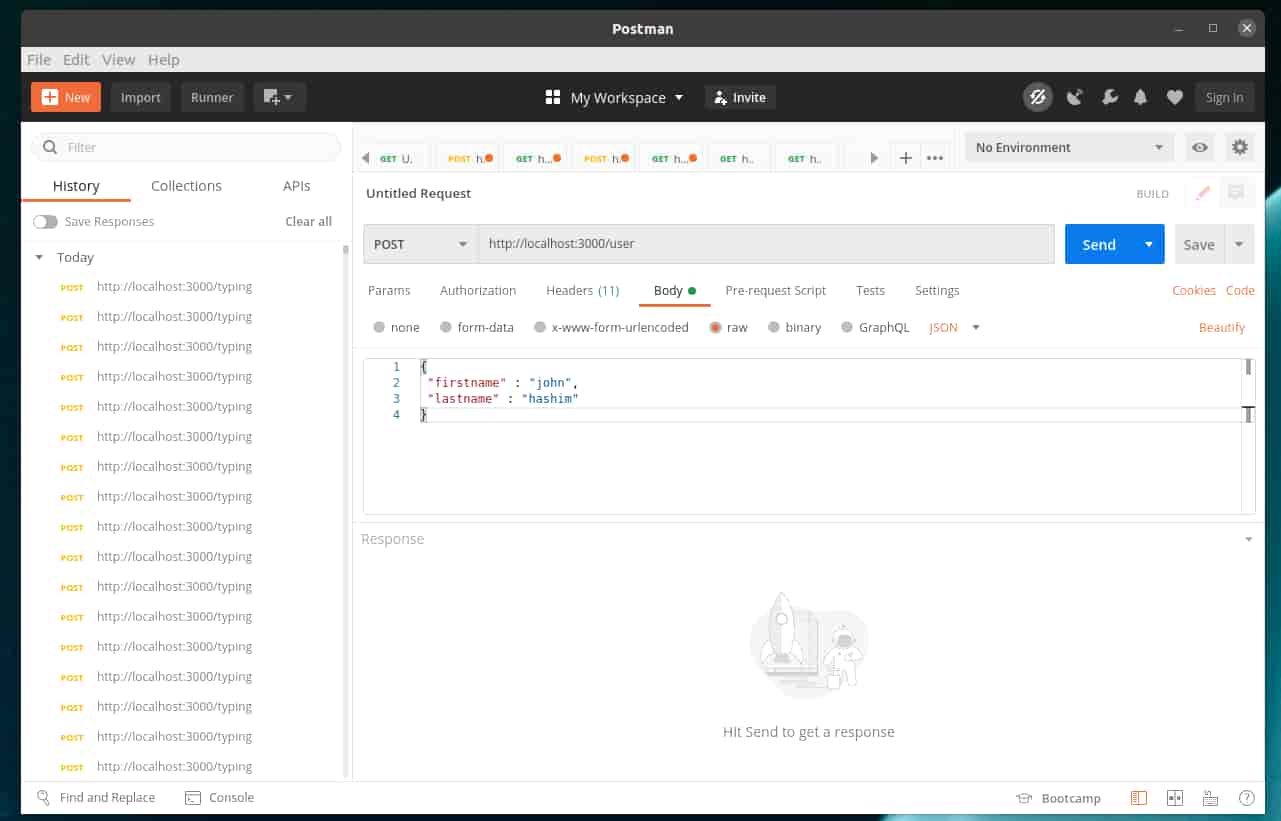
Now you shoould be able to get your data every time you send a POST request to your server.